Session 11
Simple Input Forms
Buttons & Menus
A reliable way to restrict the user's input is to offer only a variety of fixed options instead of an input field. For instance, if you use a form to ask for sampling frequency, instead of doing so with a input field like natural Sampling_frequency 44100
, you offer a range of options (e.g. 8000, 10000, 16000, 22050, 44100, 48000) for the user to choose from. This can be implemented as a set of radio buttons or as a menu. Let's do buttons first:
form Specify sampling frequency
choice Sampling_frequency_(Hz) 5
button 8000
button 10000
button 16000
button 22050
button 44100
button 48000
endform
The button set is initialized by the field type choice
, followed by the variable representation (same tricks as discussed in the previuos sections apply), followed by the default option. Default option 5 means that the fifth button (44100) is checked when the form is displayed (or when the Standards button is clicked). The resulting form:
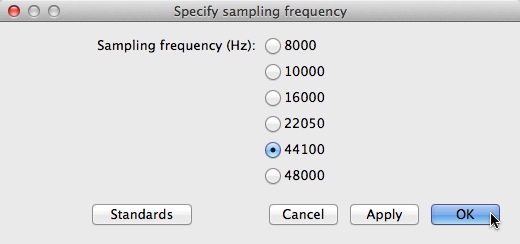
When the user chooses an option and clicks OK, the input is stored in two variables: (1) the index of the chosen option is assigned to the numeric variable sampling_frequency and (2) the content of the chosen option is assigned to the string variable sampling_frequency$. For instance, if the user selects a sampling frequency of 16000 Hz, sampling_frequency contains 3 (because 16000 is the third option) and sampling_frequency$ contains the string 16000 (coincidentally, it's a string consisting of digits, but it's a string nonetheless). If you want to use the option directly, for example to create a tone, the string has to be converted to a number (with the number ()
function):
form Specify sampling frequency
choice Sampling_frequency_(Hz) 5
button 8000
button 10000
button 16000
button 22050
button 44100
button 48000
endform
Create Sound as pure tone: "tone", 1, 0, 0.4, number (sampling_frequency$), 440, 0.2, 0.01, 0.01
Most of the time, you'll use the numeric variable and evaluate the input with a conditional (if option 1 was selected do that, if option 2 was selected do this…). The following example uses more 'typical' strings (including spaces) to illustrate that the content of options are basically strings:
form Show analysis
choice Display_analysis 1
button Spectrogram
button Pitch contour
button Intensity
button Formant tracks
endform
To save space in crowded forms, exactly the same functionality can be implemented as a menu rather than a set of buttons. Just replace the field type choice with optionmenu and button with option—that's it:
form Specify sampling frequency
optionmenu Sampling_frequency_(Hz) 5
option 8000
option 10000
option 16000
option 22050
option 44100
option 48000
endform
This produces a form, where the user can choose the sampling frequency from a pulldown menu instead of clicking buttons:
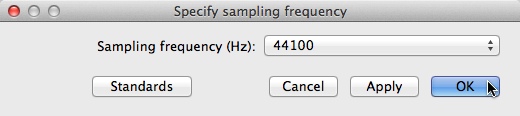
Processing is identical to the button version: The input is assigned to two variables, one numeric (index), one string (content).
Recap
Definitions of input forms are enclosed between form
and endform
. The keyword form must be followed by a space and a string, the string being the title of the form window. If a script is launched which contains a form definition somewhere, the form is always processed first.
A form definition consists of a sequence of field definitions, one field definition per line. A field definition must comply with the following pattern (except comments):
field-type variable-representation standard-value
Field-type is one of 10 available field types, variable-representations are parsed, standard values are optional.
If the field type is choice or optionmenu, options are defined with the keyword button or option, respectively, followed by a space, followed by a string. The user's choice is assigned to a numeric variable (index of option) as well as to a string variable (content of option).